AS3 sockets with C++
This short tutorial shows some sample code to get started with networking C++ and AS3. This tutorial expects that you have a basic understanding of C++, AS3, and network programming. Also this is my first tutorial.
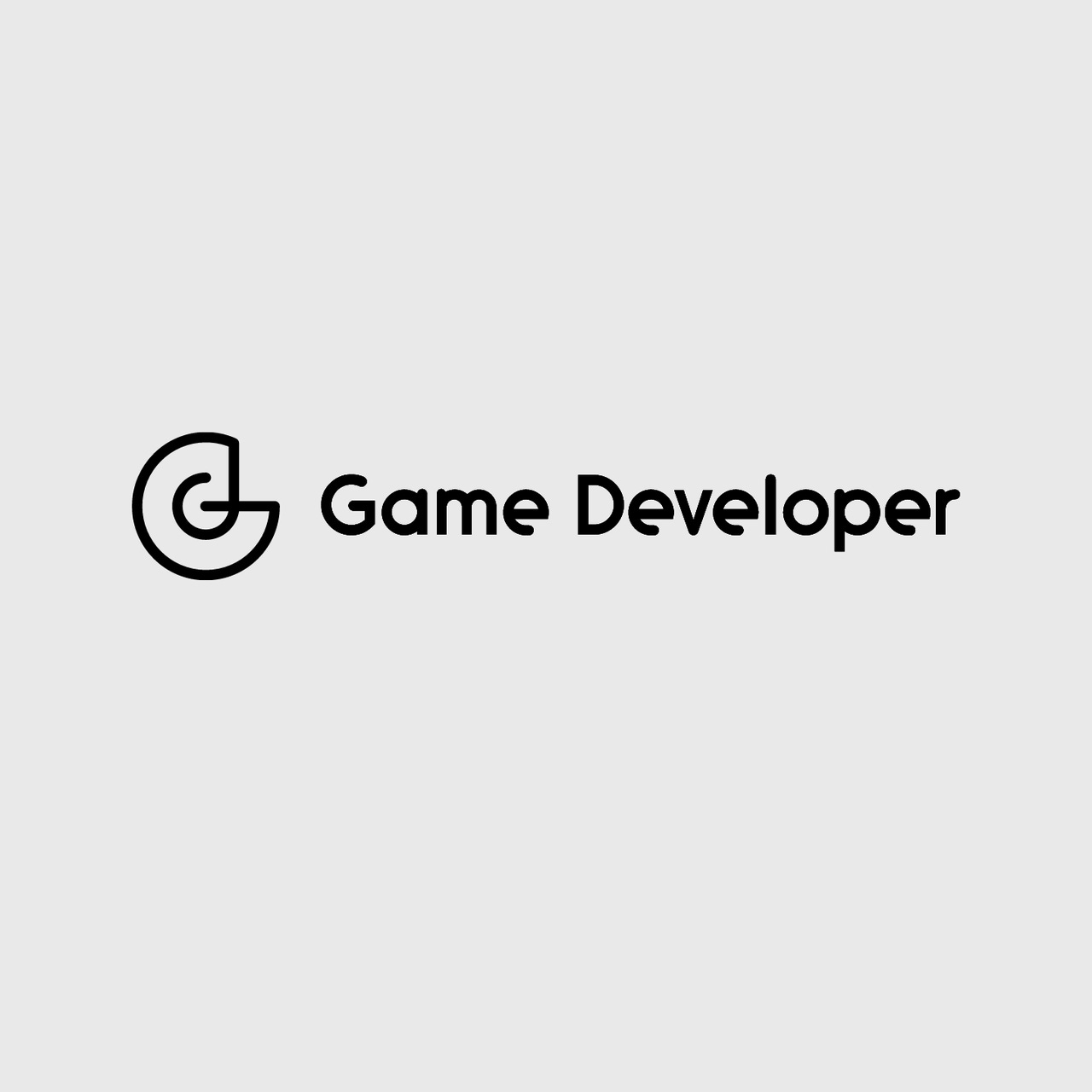
A brief description of my development environment:
Visual C++ Express 2008
Flash Develop 4.0 (I highly reccomend this as a free Flash project IDE.)
Windows 7 x64
Apache 2.2 web server
Since I have my web server on my development machine all my host connections are to "localhost"
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
AS3 code:
package
{
import flash.display.Sprite;
import flash.net.Socket;
import flash.text.TextField;
import flash.events.*;
/**
* ...
* @author Luke Manrodt
*/
public class Main extends Sprite
{
private var testText:TextField; //used to show us our connection status
private var sock:Socket;
private var host:String = "localhost";
private var port:int = 8004;
public function Main():void
{
testText = new TextField();
testText.text = "main";
testText.x = 150;
testText.y = 150;
testText.width = 600;
stage.addChild(testText);
if (stage) init();
else addEventListener(Event.ADDED_TO_STAGE, init);
}
private function init(e:Event = null):void
{
removeEventListener(Event.ADDED_TO_STAGE, init);
// entry point
sock = new Socket();
sock.addEventListener(Event.CONNECT, OnConnect);
sock.addEventListener(Event.CLOSE, OnClose);
sock.addEventListener(IOErrorEvent.IO_ERROR, OnError);
sock.addEventListener(ProgressEvent.SOCKET_DATA, OnResponse);
sock.addEventListener(SecurityErrorEvent.SECURITY_ERROR, OnSecError);
sock.connect(host, port);
testText.text = "connecting to "+host;
}
public function OnConnect(e:Event = null):void
{
testText.text = "connected to "+host;
}
public function OnClose(e:Event = null):void
{
testText.text = "connection closed";
sock.connect(host, port);
}
public function OnError(e:Event = null):void
{
testText.text = "IO error";
}